Project duration: 1 week
Project type: individual (but feel free to help each other)
The objective in this project is to decode a secret 4-character message that has been encoded as an optical binary signal in the form of a flashing region in a video. Each of you has been assigned a unique 4-character code. You will build a simple optical detector circuit that can be used to record the binary signal and display it on the oscilloscope so that you can decode the secret message.
Alternative link to download video file
- Each character is encoded as a single byte (8 bits) using the ASCII system.
- Each byte is transmitted least significant bit (lsb) first.
- Each byte is preceded by a start bit (equal to 1).
- Each byte is followed by a stop bit (equal to 0).
- Bits equal to “1” are represented by white in the video.
- Bits equal to “0” are represented by black in the video.
The following example signal encodes the word “Demo”:
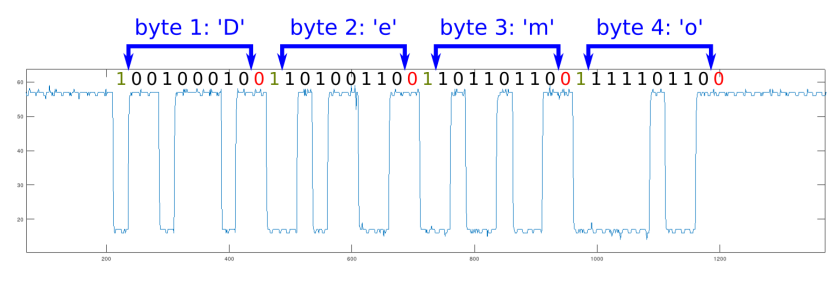
Instructions
- Build a photodetector circuit (two options are provided below – version 2 is recommended).
- Use an oscilloscope to check that the output voltage of the photodetector changes when the intensity of light falling on the LED(s) changes.
- Identify the flashing region labelled with your initials in the binary signal video.
- Hold the LED(s) up to that part of the screen and use the oscilloscope to record the binary waveform. Once you have the complete 4-byte transmission displayed on the oscilloscope screen, press pause so that you can photograph it and decode the signal.
- Once you’ve figured out your individual 4-character secret message, check with your lab supervisor to see if you got it right.
- ADVANCED CHALLENGE: If you complete all previous parts, try programming the Arduino to automatically decode this type of binary signal and print the 4-character code word in the Serial Monitor. Feel free to collaborate with your classmates on this part.
Submit a 1-page Word document to Brightspace containing the following:
- Your name.
- Your individual 4-character secret message.
- A photograph of your photodetector circuit.
- A photograph of your complete 4-byte binary transmission displayed on the oscilloscope screen.
- OPTIONAL: If you attempted the advanced challenge, include evidence of whatever you did in the Word document.
Photodetector circuit
Two photodetector circuits are provided below. The first is simpler and easier to understand, but the second one works much better. You are advised to use the second one for this challenge.
Version 1 : Simple but flawed
- Minimal design.
- Easy to build and understand.
- Works, but not very well.
- The reverse-biased LED acts as a photodiode. When light strikes it, a tiny reverse current is allowed to pass through it. The more intense the light, the larger the current (although it’s always very small).
- The NPN bipolar junction transistor (BJT) acts as a current amplifier. The tiny base current arriving from the LED controls a much larger collector current, which passes through the resistor R.
- The voltage across resistor R varies with the magnitude of the collector current, and hence with the intensity of light falling on the LED.
Version 2 : Much improved output signal
- Same principle of operation as the previous circuit.
- The addition of a second LED results in twice as much current for the same light intensity.
- The two transistors are connected as a Darlington pair, which results in an overall current gain that is approximately equal to the product of the individual transistors’ current gains.
- This circuit produces a much clearer voltage output signal than the other version of the circuit.
- A smaller resistor can be used because the increased current gain results in a larger collector current. Reducing the resistance allows the output voltage to respond faster to changes in light intensity.
Alternative method using Arduino instead of oscilloscope
To complete the binary signal detective challenge without an oscilloscope, the Arduino Nano and Serial Plotter can be used to visualise the binary waveform output from the photodetector circuit. Just upload the following code to the Arduino and then connect the output of the photodetector circuit to pin A0.
void setup()
{
Serial.begin(9600);
}
void loop()
{
Serial.print("0 1023 ");
Serial.println(analogRead(0));
delay(20);
}
Open the Serial Plotter (under the Tools menu in the Arduino development environment) and you should see a waveform resembling the one below when you hold your LED photodetectors up to the flashing signal on the screen.
